Here’s the prompt I gave ChatGPT:
Draw a Christmas tree using SwiftUI
Here’s the code I got:
struct ChristmasTree: View {
var body: some View {
ZStack {
// draw the tree trunk
Rectangle()
.fill(Color.brown)
.frame(width: 20, height: 100)
// draw the tree branches
ForEach(0..<5) { i in
HStack(spacing: 5) {
ForEach(0..<5-i) { j in
Triangle()
.fill(Color.green)
.frame(width: 10, height: 10)
}
}
.offset(x: 0, y: -10 * CGFloat(i))
}
// draw the star on top of the tree
Circle()
.fill(Color.yellow)
.frame(width: 10, height: 10)
.offset(x: 0, y: -50)
}
}
}
The only major issue syntax-wise is that Triangle() isn’t defined – where did it get that?
A quick visit to Hacking with Swift had this nice snippet, which I dropped in.
struct Triangle: Shape {
func path(in rect: CGRect) -> Path {
var path = Path()
path.move(to: CGPoint(x: rect.midX, y: rect.minY))
path.addLine(to: CGPoint(x: rect.minX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.midX, y: rect.minY))
return path
}
}
Now we can compile and…. tada!
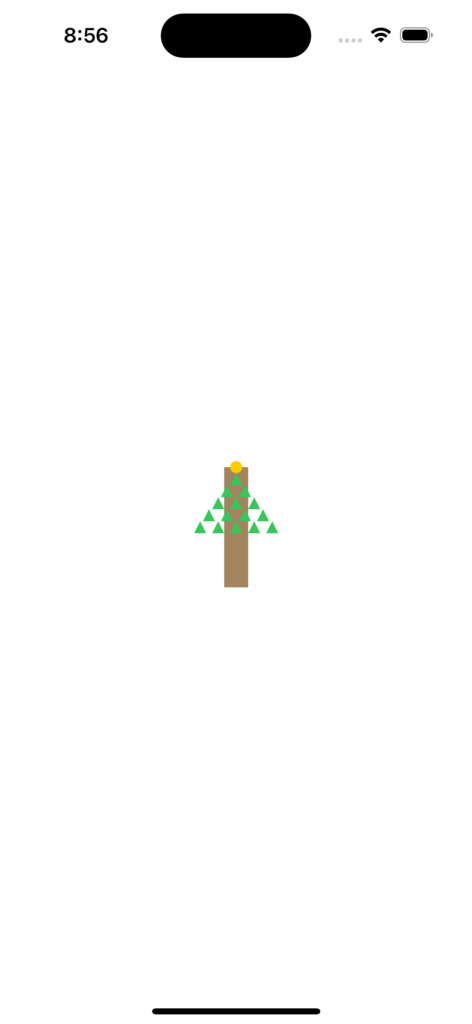
Not great, but darn impressive! 🎄🤯